Unity iOS - How to Disable Swizzling
Leanplum comes with out of the box swizzling and notifications setup. There are cases where this could conflict with other libraries or your native code. In such cases, swizzling can be disabled and Leanplum methods to be called manually, as described in this article.
Extend the UnityAppController
In Unity, the UnityAppController serves as the AppDelegate. It should be extended with the logic for push notifications.
Example:
//
// LeanplumUnityAppController.m
// UnityFramework
//
// Created by Nikola Zagorchev
//
#import <Foundation/Foundation.h>
#import <UserNotifications/UserNotifications.h>
#import <Leanplum/Leanplum.h>
#import "UnityAppController.h"
@interface LeanplumUnityAppController : UnityAppController<UNUserNotificationCenterDelegate>
@end
@implementation LeanplumUnityAppController : UnityAppController
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary<UIApplicationLaunchOptionsKey,id> *)launchOptions
{
BOOL result = [super application:application didFinishLaunchingWithOptions:launchOptions];
[[UNUserNotificationCenter currentNotificationCenter] setDelegate:self];
[Leanplum applicationDidFinishLaunchingWithOptions:launchOptions];
return result;
}
- (void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo fetchCompletionHandler:(void (^)(UIBackgroundFetchResult))completionHandler
{
[Leanplum didReceiveRemoteNotification:userInfo];
completionHandler(UIBackgroundFetchResultNewData);
}
- (void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken
{
[Leanplum didRegisterForRemoteNotificationsWithDeviceToken:deviceToken];
}
- (void)application:(UIApplication *)application didFailToRegisterForRemoteNotificationsWithError:(NSError *)error
{
[Leanplum didFailToRegisterForRemoteNotificationsWithError:error];
}
- (void)userNotificationCenter:(UNUserNotificationCenter *)center didReceiveNotificationResponse:(UNNotificationResponse *)response withCompletionHandler:(void (^)(void))completionHandler
{
[Leanplum didReceiveNotificationResponse:response];
completionHandler();
}
-(void)userNotificationCenter:(UNUserNotificationCenter *)center willPresentNotification:(UNNotification *)notification withCompletionHandler:(void (^)(UNNotificationPresentationOptions options))completionHandler
{
[Leanplum willPresentNotification:notification];
completionHandler(UNNotificationPresentationOptionNone);
}
@end
IMPL_APP_CONTROLLER_SUBCLASS(LeanplumUnityAppController)
Put the file into Assets -> Plugins -> iOS so it is copied automatically to the iOS Project.
Note that now you need to add the UserNotifications framework to the UnityFramework project.
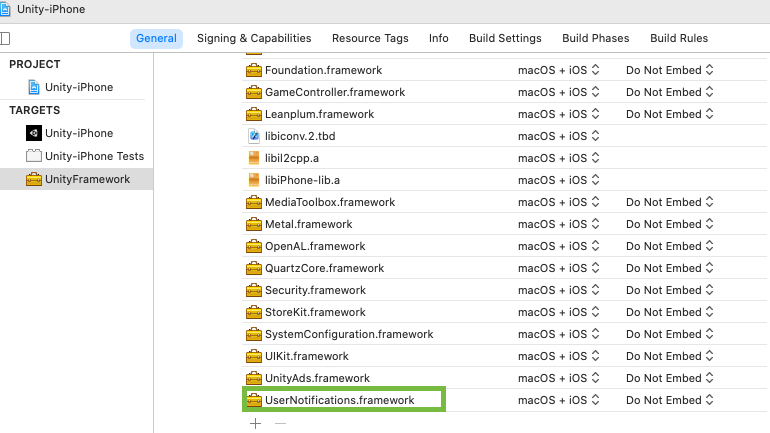
Set the flag in Info.plist
Now the Info.plist file needs to be updated to mark that swizzling is disabled. Set a boolean flag for LeanplumSwizzlingEnabled
to NO
.
This can also be done directly from Unity using a PostProcessBuild
task.