Quickly start up your iOS integration with Leanplum
This article is your first step to activating the Leanplum iOS SDK. Once completed, you will have access to all the goodness Leanplum provides. Follow the steps below.
STEP 1: Install the SDK
To install the Leanplum SDK there are two main options, downloading Cocoapods or through a manual setup.
Supports iOS 9.0 and above
A. Download Cocoapods and Add to Podfile (recommended)
- If you don't already have CocoaPods installed and a Podfile in your project, run the following script in Terminal
sudo gem install cocoapods
pod init
open -a Xcode Podfile
- Once downloaded, add the following to your Podfile
pod 'Leanplum-iOS-SDK', '<<LEANPLUM_IOS_SDK_VERSION>>'
# Uncomment one of the following for Location Services.
# pod 'Leanplum-iOS-Location', '<<LEANPLUM_IOS_SDK_VERSION>>'
# pod 'Leanplum-iOS-LocationAndBeacons', '<<LEANPLUM_IOS_SDK_VERSION>>'
- Finally, run the below command to install the Leanplum SDK to your project
pod install
- Xcode 14 requires codesigning for bundles, you can override this by using the following
post_install
script
post_install do |installer|
installer.pods_project.targets.each do |target|
if target.respond_to?(:product_type) and target.product_type == "com.apple.product-type.bundle"
target.build_configurations.each do |config|
config.build_settings['CODE_SIGNING_ALLOWED'] = 'NO'
end
end
end
end
- If you are using Xcode 15, set
ENABLE_USER_SCRIPT_SANDBOXING
toNO
in the Build Settings.
B. Install through Swift Package Manager
- You can install the Leanplum SDK through SPM by using the package URL which is the GitHub repo:
https://github.com/Leanplum/Leanplum-iOS-SDK
Note that SPM uses a binary target since the Leanplum SDK uses mixed languages.
-
Once you have installed the package, ensure you have the
-ObjC
flag in Linking -> Other Linker Flags. You might also need to add the-all_load
flag. -
Add the following script to the app Build Phases -> New Run Script Phase:
cp -R -L ${BUILT_PRODUCTS_DIR}/Leanplum.framework/*.bundle ${BUILT_PRODUCTS_DIR}/${TARGET_NAME}.app
The script ensures that the Leanplum Resources bundle is available in the app.
Note that if Leanplum is packaged as part of another framework, the above script needs to be modified to target the framework containing Leanplum. Example where YOUR_FRAMEWORK is the name of the framework containing Leanplum:
cp -R -L ${BUILT_PRODUCTS_DIR}/Leanplum.framework/*.bundle ${BUILT_PRODUCTS_DIR}/${TARGET_NAME}.app/Frameworks/YOUR_FRAMEWORK.framework
- If you are using Xcode 15, set
ENABLE_USER_SCRIPT_SANDBOXING
toNO
in the Build Settings.
Leanplum SDK 6.0.0 and above
Uses the static xcframework for the binary.
The Package 'wraps' the Leanplum binary target, so dependencies can be added - in this case, the CleverTap SDK. SPM will install three packages - Leanplum SDK (binary), CleverTap SDK (source, dependency), SDWebImage (source, dependency).
Leanplum SDK 5.0.0 and bellow
Uses the dynamic xcframework for the binary.
Older versions before that, which were Objective-C only, use the source code directly.
C. Manually Install iOS SDK
If you would rather like to manually install our iOS SDK, please use the following steps
- Visit and download the latest release of our SDK from Github
We provide static and dynamic xcframeworks.
- Add the Leanplum.xcframework to Frameworks, Libraries and Embedded Content in your project.
Leanplum iOS SDK 6.0.0 and above requires the CleverTap SDK and its dependencies to be installed.
The dynamic xcframework does not include the dependencies since umbrella frameworks are discouraged by Apple and no longer pass AppStore validation.
The static xcframework does not include it, to prevent duplicate symbols. In this case, you need to manually install the CleverTap SDK and its dependencies.
NOTE: If you require to use Location services on Leanplum, add LeanplumLocation.xcframework
or LeanplumLocationAndBeacons.xcframework
if you need iBeacon services.
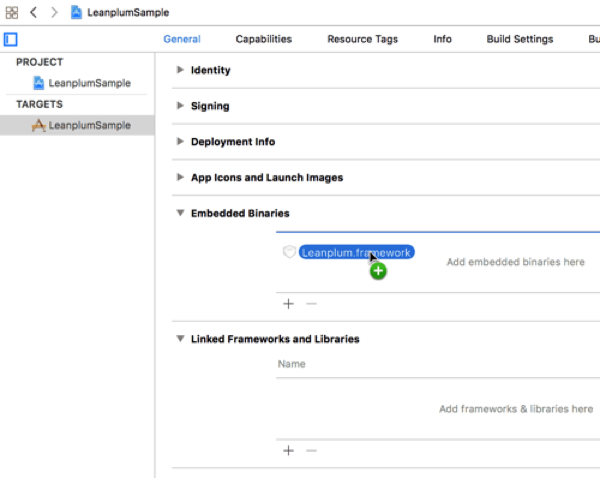
-
Choose “Embed & Sign” for dynamic xcframework, and “Do not embed” for the static one.
-
Add the following Frameworks to your project
CoreLocations.framework
CFNetwork.framework
SystemConfiguration.framework
Security.framework
AdSupport.framework
StoreKit.framework
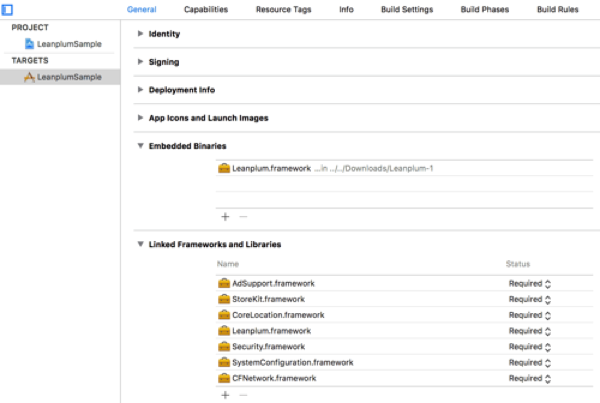
- Additional Configurations:
Static:
Add the following script to the app Build Phases -> New Run Script Phase:
cp -R -L ${BUILT_PRODUCTS_DIR}/Leanplum.framework/*.bundle ${BUILT_PRODUCTS_DIR}/${TARGET_NAME}.app
The script ensures that the Leanplum Resources bundle is available in the app.
Dynamic:
When using dynamic frameworks, you can use the xcframeworks of CleverTapSDK and SDWebImage included in the Leanplum.zip for each release.
STEP 2: Import Leanplum Into Your App Delegate
Below is sample code you can use in your App Delegate to get Leanplum started. For more on API Keys visit our article on our API keys.
import UIKit
#if DEBUG
import AdSupport
#endif
import Leanplum
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(application: UIApplication!, didFinishLaunchingWithOptions launchOptions: NSDictionary!) -> Bool {
// You will find your API Keys at https://www.leanplum.com/dashboard?#/account/apps
#if DEBUG
Leanplum.setDeviceId(ASIdentifierManager.shared().advertisingIdentifier.uuidString)
Leanplum.setAppId("YOUR_APP_ID",
withDevelopmentKey:"YOUR_DEVELOPMENT_KEY")
#else
Leanplum.setAppId("YOUR_APP_ID",
withProductionKey: "YOUR_PRODUCTION_KEY")
#endif
// Sets the app version, which otherwise defaults to
// the build number (CFBundleVersion).
Leanplum.setAppVersion("YOUR_APP_VERSION")
// Starts a new session and updates the app content from Leanplum.
Leanplum.start()
return true
}
#import "AppDelegate.h"
#import <Leanplum/Leanplum.h>
@implementation AppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
/// You will find your API Keys at https://www.leanplum.com/dashboard?#/account/apps
#ifdef DEBUG
[Leanplum setAppId:@"YOUR_APP_ID"
withDevelopmentKey:@"YOUR_DEVELOPMENT_KEY"];
#else
[Leanplum setAppId:@"YOUR_APP_ID"
withProductionKey:@"YOUR_PRODUCTION_KEY"];
#endif
// Optional: Tracks in-app purchases automatically as the "Purchase" event.
// To require valid receipts upon purchase or change your reported
// currency code from USD, update your app settings.
// [Leanplum trackInAppPurchases];
// Sets the app version, which otherwise defaults to
// the build number (CFBundleVersion).
[Leanplum setAppVersion:@"2.4.1"];
// Starts a new session and updates the app content from Leanplum.
[Leanplum start];
return YES;
}
...
@end
Setting AppIds from plist file
Starting from iOS SDK 3.1.0, we support the option to provide the AppId, Production, and Development keys in a plist file. If the keys are not set before calling Leanplum.start they are loaded from the Leanplum-Info.plist if such exists.
Example:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>APP_ID</key>
<string>app_key</string>
<key>DEV_KEY</key>
<string>dev_key</string>
<key>PROD_KEY</key>
<string>prod_key</string>
<key>ENV</key>
<string>development</string>
</dict>
</plist>
The Environment key (ENV) value must be either development or production. The environment can also be changed using Leanplum setAppEnvironment method which accepts the same values.
Use Your Development Key Only During Your Development Phase
The Leanplum development key leverages open socket technology in our development pipeline for real-time previewing and analytics. This pipeline therefore cannot support a live production scale and any user data maybe lost as it is not captured in analytics.
Additionally for tighter security, make sure to remove your development key from your app delegate before submitting to the App Store.
STEP 3: Run Your Build
Build and run your app and see data start flowing into Leanplum. With your development key in your app delegate, you will be able to see all data flowing into Leanplum in our debugger. You can access our debugger dashboard at https://leanplum.com/dashboard#/help/debug
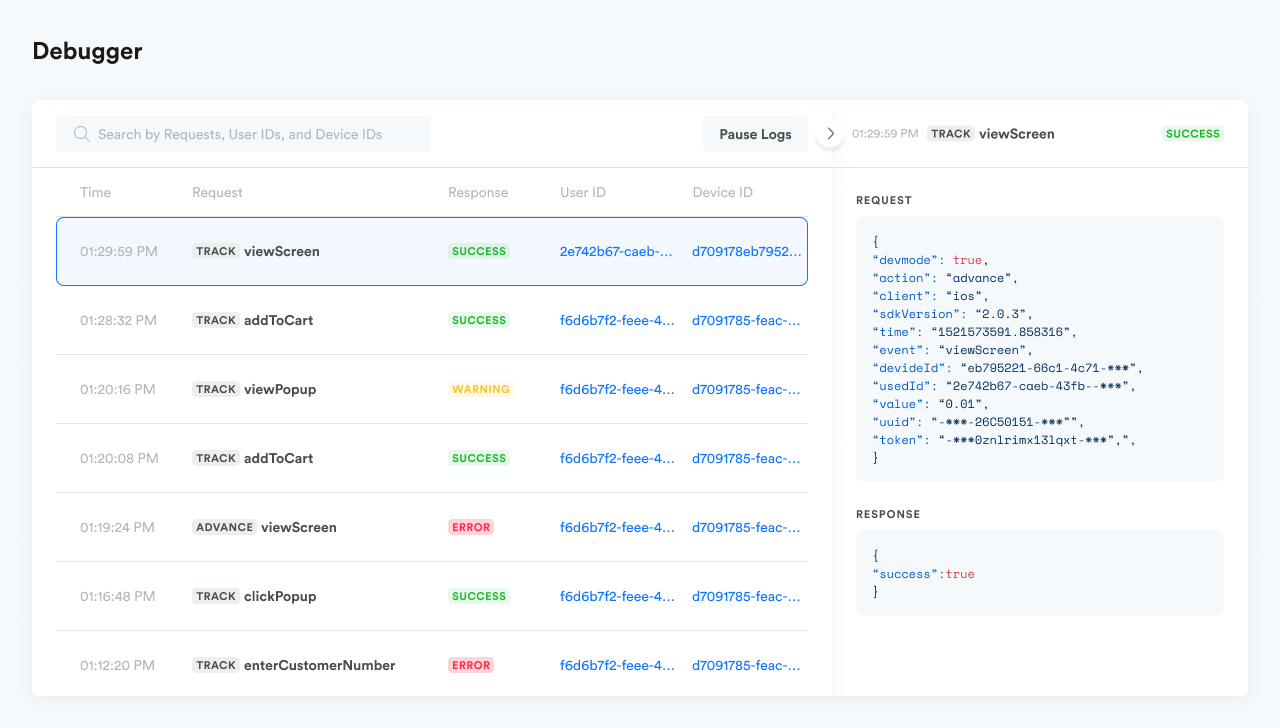
App Version
The App Version that will be captured in Leanplum when running your build in iOS will default to the main bundle CFBundleVersion when called.
STEP 4: Register Your Test Device
Registering your device as a test device will allow you to preview your messages, variable changes, and any other Leanplum projects. Follow the below to register.
1. Enable the DEBUG preprocessor macro in your Build Settings
Registering a test device will allow you to test your messages, variable changes, and other Leanplum projects on a real device.
To register your device, first make sure you're in Debug mode. To ensure Debug mode is enabled, make sure the DEBUG preprocessor macro is set in Build Settings.
Apple Clang - Preprocessing -> Preprocessor Macros -> Debug. Set value to DEBUG=1.
Add DEBUG flag (Swift only)
In your Build Settings, add -D DEBUG to Debug mode in Other Swift Flags.
2. Register Your Device On the Test Devices Page
Visit https://leanplum.com/dashboard#/account/devices and click on "Register test device" to register your device
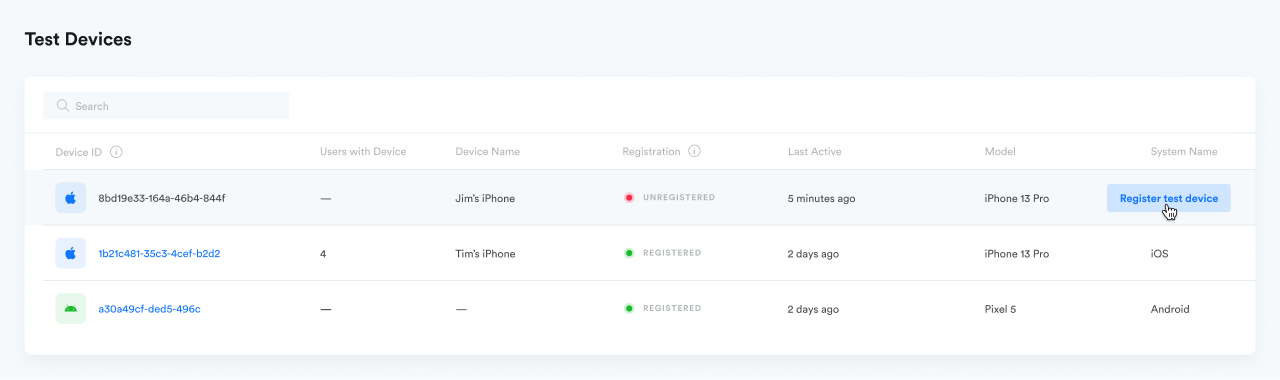
STEP 5: Complete Your Integration (NEXT STEPS)
Success! You have now successfully installed the Leanplum iOS SDK and registered a test device for further testing. This is an exciting first step on the journey to full integration with Leanplum.
To complete your integration with Leanplum, check out the below articles to learn more about your user data, tracking with Leanplum, variables, importing data and setting up messages.
Articles to Visit Next
- Collecting User Data
- Event Tracking
- State Tracking
- Variables
- Importing Data
- Setting Up Push Notifications
- Build Custom In-App Messages