Handling logins with UserIDs
This article explains how Leanplum creates User Profiles and how to handle UserIDs when your users log in after Leanplum.start is called. When Leanplum starts for the first time and a new user profile gets created, the UserID value is set as identical to the DeviceID value by default. Then Leanplum starts tracking sessions for this user.
Device IDs in Leanplum
The Device ID uniquely identifies the device and is determined automatically by the SDK.
- On iOS, by default, we use the identifierForVendor (IDFV). If the device is pre-iOS 6, we use the hash of the MAC address, and in development mode, we use the advertising identifier.
- On Android, by default, we use the ANDROID_ID. If the device is pre-Lollipop and
android.permission.ACCESS_WIFI_STATE
is set, we use the MD5 hash of the MAC address, otherwise we use ANDROID_ID.
See our docs under User and device tracking for more details.
Using setUserId for logins and user profies
This is important when developing the user flow logic of your app — here's a login flow for an app where the login (setUserId) occurs after the Leanplum.start call:
-
Register/first login: The first time
setUserID(“NewCustomUserID”)
is called, the ‘NewCustomUserID’ string is passed as the User ID, which will replace the existing User ID value in the same User Profile. (The existing User ID was previously identical to the Device ID, but now it will be ‘NewCustomUserID’.) The user's profile will maintain all the data tracked before the login, including the Device ID. -
Different login, same device: From now on, every time setUserID is called, passing another string value, a new user profile will be created. The current user's session will be closed, and another session will be opened for the new user that was just passed as a string. If the new User ID doesn’t exist, a new User Profile will be created.
Setting userID with Leanplum.start
You can also pass a custom User ID value as part of the Leanplum.start()
parameters, but this may not be the best way to do it if you plan on having users log in later.
If you set the User ID through
Leanplum.start
, then callsetUserID
again later for a login or other scenario, Leanplum will close the current User ID session and start logging a new session for a new user. If the user doesn't exist yet, a new profile will be created for them.
Should I set my userIDs with Leanplum.start() or use setUserId later on?
Using setUserId
is best when a new user installs the app for the first time and starts playing around with it, then logs in sometime after Leanplum.start
has already taken place. This will ensure that any activity from that user before they logged in will stay in that user's profile. In this scenario, calling setUserId
again with a different string value means that another user has logged in — this will close the previous session and start a new session for the latest User passed in the setUserId parameter.
Using Leanplum.start()
will create a new user profile on start. This means that if you call setUserId again after start
, Leanplum will assume this is a different user, ending the original session for the first user and starting a new one for the new user.
Do not hard code a User ID for multiple users
Do NOT use
setUserId
for representing a LOGOUT scenario, e.g. don't set a userId = "LOGGED_OUT_USER" for many users. Instead, we suggest using asetUserAttribute
flag to indicate that the user is logged out. Leanplum will always be in execution and tracking sessions for the last user who has been passed in asetUserId
.
Sample
Take a look at some sample code for more details:
When the example is run for the first time, the DeviceID value taken by default is going to be also the value used for the User Profile UserID when creating a new User Profile.
The DeviceID can be customized, but Leanplum.setDeviceID has to be run before Leanplum.start() - also beware that a DeviceID can be set only when starting the app for the first time and from a new installation, otherwise it would do nothing (i.e. you cannot change the DeviceID value if the app is already installed and run at least once).
1. Fill App keys in the sample and start the app. Check the Leanplum Dashboard.
Result: Assuming this is the first time you run the sample, you will notice a new User Profile is created and the UserID si set identical by default to the on the DeviceID value:
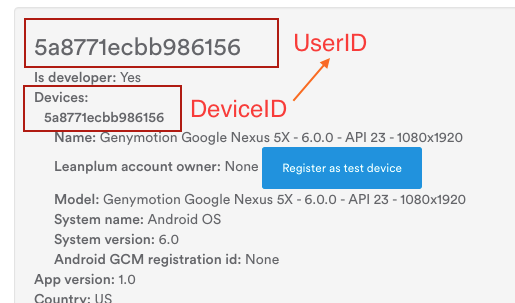
2. On the Device, fill in some text representing a new UserID for logging in, and click on the login button.
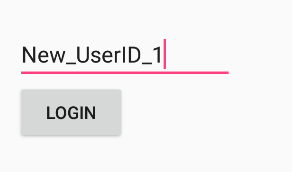
When you click the Login button, the code is getting the String value and passing it inside setUserID
method:
// iOS
[Leanplum setUserId:<login_field_string>];
// Android
Leanplum.setUserId(<login_field_string>);
3. Check Dashboard and console log.
Assuming this was the first time and the User Profile was created first time in bullet #1, you will notice that the new UserID is being merged in the existing User Profile, taking place of the latest UserID value (identical to the deviceID). All the user data collected before the login are maintained.
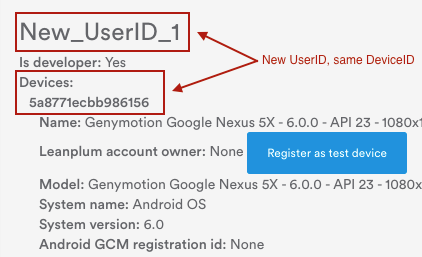
Basically, so far we described the scenario of a new user installing the app for the first time, playing around with it and then she decides to login specifying a UserID. We store all the collected data so far and change the UserID from the DeviceID value to the newly passed UserID value
4. Click on Logout button.
Result: the current activity is closed but we don't pass any specific UserID value for representing the logout user action. Leanplum will always be in execution and tracking data for the last User who has been passed in the SetUserID. We can use a userAttribute to flag that the User is logged off, in case needed for sorting those users out or for segments.
5. Then click on the Login button again passing another UserID value.
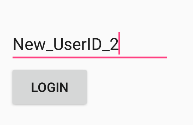
6. Check Dashboard and console log.
This time a new User Profile is going to be created.
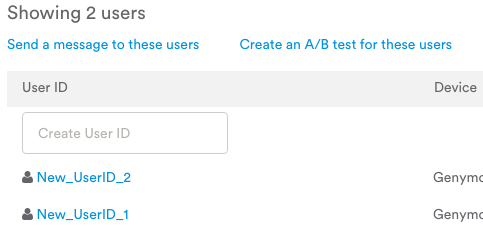
We already logged in with another user before, so in this case the session for the previous user is going to be closed and a new User Profile is going to be created with the new UserID passed in the new login. The previous User session is closed and new session is started for the new User.
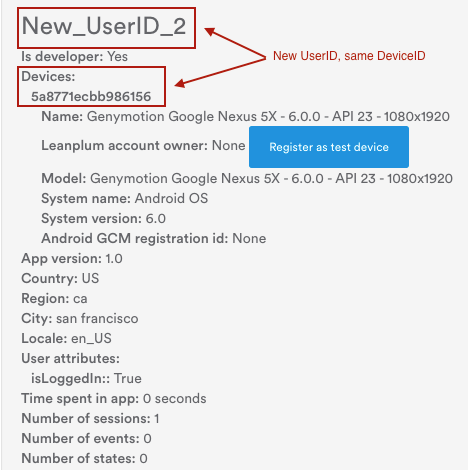
This reflects the case of a New User who is logging in into the same device, therefore what we want to happen is to track everything that follows as a new user, separate from the first one who launched the app first time and then logged in.
Also note the results when searching now for one of the UserIDs or for the DeviceIDs. In case of searching for a specific userID, only that User is going to be found in the results:
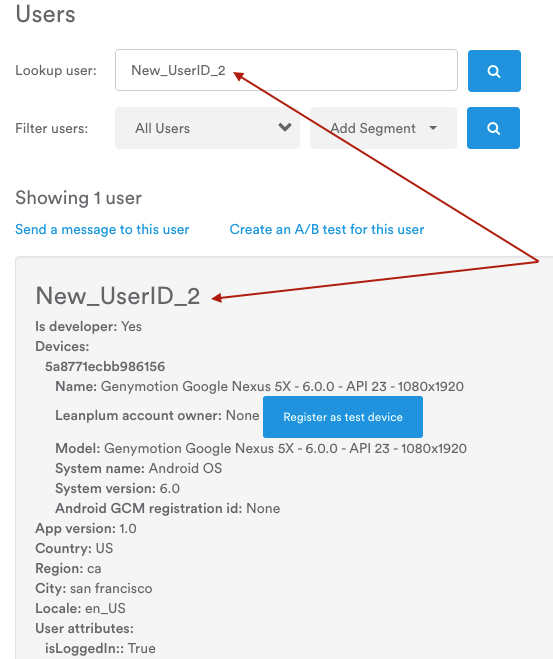
However, searching for the DeviceID being used for those cases, all the User profiles including that DeviceID are going to be displayed:
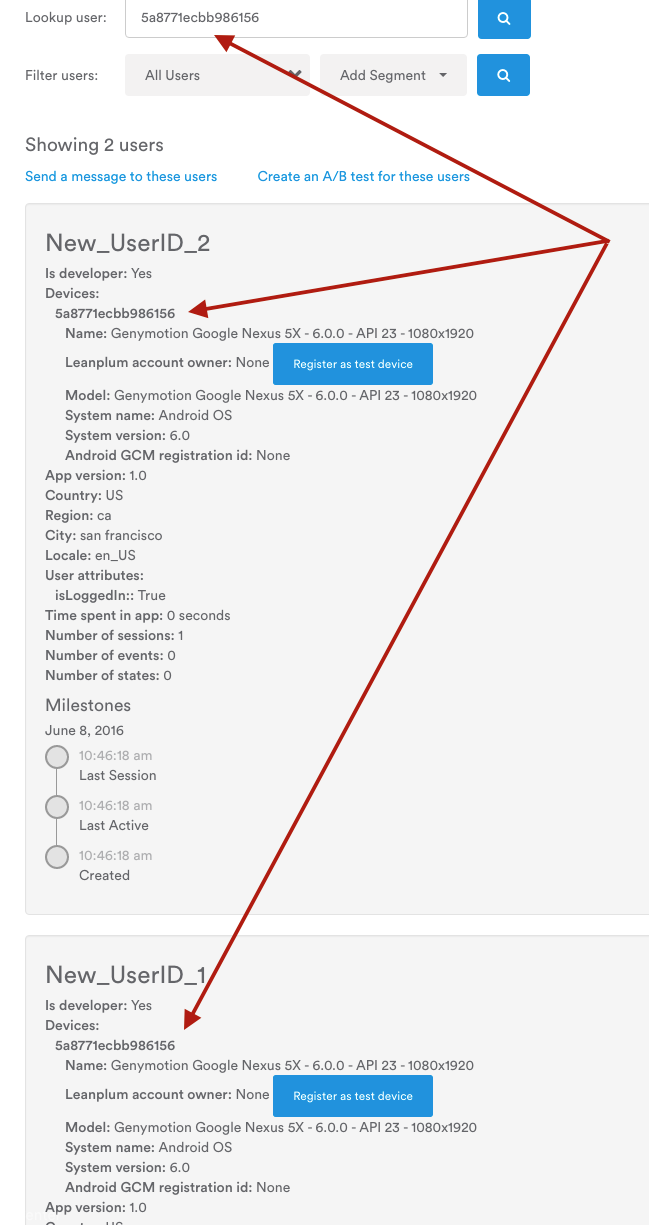
Updated over 4 years ago