Instrumenting variables
Set up your application to use variables that are controlled from the Leanplum dashboard
By using Leanplum Variables, after an initial setup, you can roll out changes to the application without development effort.
Variables also allow you to perform an A/B test with a percentage of your users.
Prerequisites:
- You must have a registered test device. For information, see Register your test device.
To set up your app to work with Leanplum Variables:
-
Define the Variables in your application.
Variables can be of different types — Integer, Boolean, String, Dictionary, File. To define a variable on a specific platform, see the platform-specific documentation below. -
Run the app in development mode on your test device
-
Verify that the variables defined on the client are visible on the Variables page.
For example:
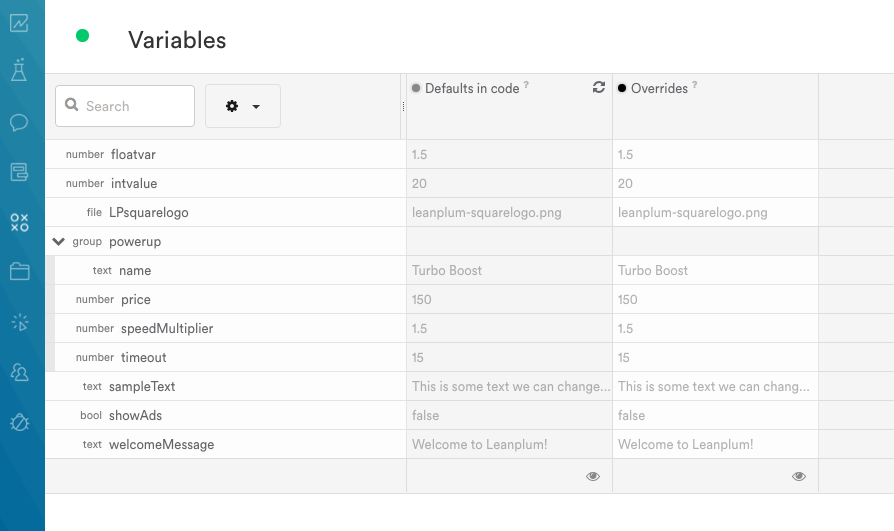
- Access the Variable values in your code.
Variables are retrieved asynchronously
After your app starts, the Leanplum SDK retrieves the Variable values asynchronously. When using Variables, your application should wait until the Variable values are retrieved from the server.
iOS
You can access the iOS sample code in the iOS samples repository.
Defining variables
On iOS with ObjectiveC, you define variables in any class of your code, like you would a constant (outside methods).
Use the DEFINE_VAR macros to grab all Variables as soon as the app starts.
In this sample we define Variables both in the AppDelegate.m and in ViewController.m using the DEFINE_VAR macros to define different kind of variables. In this case, these are String, Boolean, float, int, a dictionary, and a file.
For example, in AppDelegate.m
:
DEFINE_VAR_STRING(welcomeMessage, @"Welcome to Leanplum!");
DEFINE_VAR_BOOL(showAds, false);
DEFINE_VAR_FLOAT(floatvar, 1.5);
DEFINE_VAR_INT(intvalue, 20);
DEFINE_VAR_DICTIONARY_WITH_OBJECTS_AND_KEYS(
powerup,
@"Turbo Boost", @"name",
@150, @"price",
@1.5, @"speedMultiplier",
@15, @"timeout",
nil);
or in another class like ViewController.m
:
DEFINE_VAR_FILE(LPsquarelogo, @"leanplum-squarelogo.png");
When the project launches in Development mode and the device is registered, all the Variables that you define in the project by using the DEFINE_VAR macros are synchronized and added to the Dashboard.
Using callbacks
When the app starts, the SDK retrieves Variable data asynchronously. To use the latest values of the variables, use Variables inside Leanplum callbacks.
Following the sample project code, once the app starts, callbacks are triggered based on when variables values are changed. In this case, their values are printed in the console:
[Leanplum onVariablesChanged:^{
NSLog(@"%@", welcomeMessage.stringValue);
NSLog(@" %s", showAds.boolValue ? "true" : "false");
NSLog(@"%0.1f", floatvar.floatValue);
NSLog(@"%d", intvalue.intValue);
NSLog(@"%2f", [[powerup objectForKey:@"speedMultiplier"] floatValue]);
}];
In case of files, the callback is slightly different and is triggered when the file is downloaded from the server.
For example, in the ViewController.m
class, we set the image only when no downloads are pending and the image is received:
[Leanplum onVariablesChangedAndNoDownloadsPending:^{
LPlogo.image = LPsquarelogo.imageValue;
}];
See the Sample project for more details.
Android
Defining Variables
In an Android project, Variables can be defined using an @Variable
annotation, or using Var.define
.
Using @Variable annotation
You can define these variables outside of the onCreate() method:
- Inside your Application class that extends LeanplumApplication.
- In your main activity that extends one of the LeanplumActivity classes and calls Leanplum.start
- In another class. Use the Parser class to detect the annotations before calling Leanplum.start
Using Var.define
- Anywhere in your Class before starting Leanplum.
- In another class outside of the onCreate() method. Use also in this case the Parser class to detect the variable before calling Leanplum.start
In this sample, we define different variables in different Activities and in the Application class as well.
When the App starts, the code is executed in the Application class, as well as in the MainActivity. The Variables defined in the other classes are being parsed inside the onCreate() method before Leanplum.start
executes.
In the sample, we start Leanplum in the Application class, which is also not extending a LeanplumApplication class but we are implementing the Session Lifecycle manually.
For example:
@Override
public void onCreate() {
super.onCreate();
Leanplum.setApplicationContext(this);
Parser.parseVariables(this);
Parser.parseVariablesForClasses(AnotherActivity.class, AnotherLPactivity.class);
LeanplumActivityHelper.enableLifecycleCallbacks(this);
}
Other variables are defined in the Application class itself before Leanplum start. See the Sample project and check all Activities.class
instances for an example.
Using callbacks
Once the Variables are synchronized, they trigger callbacks to notify the application. You can place callbacks where you need to use the Variable values.
In the project sample code, we are printing out the Variable values defined in the Application class.
So, for example, in the Application class:
Leanplum.addVariablesChangedHandler(new VariablesChangedCallback() {
@Override
public void variablesChanged() {
Log.i("#### ", welcomeLabel.value());
for (Map.Entry<String, Object> entry : powerup.entrySet()) {
String key = entry.getKey();
Object value = entry.getValue();
Log.i("#### ", "Application class var : " + key + " " + value.toString());
}
}
});
In the MainActivity class (accessing the Variables defined in the Application class):
Leanplum.addVariablesChangedHandler(new VariablesChangedCallback() {
@Override
public void variablesChanged() {
welcomeMessageText1.setText(ApplicationClass.String_Welcome1);
welcomeMessageText2.setText(ApplicationClass.String_Welcome2);
}
});
For images, make sure that the image value is used after it has been downloaded. In that case, you can use Leanplum.addVariablesChangedAndNoDownloadsPendingHandler
.
See the Sample project for more details.
Web / JavaScript
Since JavaScript is a dynamic language, you can set up the Variables with a JSON object, without explicitly defining their types.
Defining Variables
To define the Variable defaults, you can use the setVariables
method. Nested objects become dictionaries in the Leanplum dashboard.
Leanplum.setVariables({
welcomeMessage: "Welcome to Leanplum!",
showAds: false,
floatvar: 1.5,
intvalue: 20,
powerup: {
name: "Turbo Boost",
price: 150,
speedMultiplier: 1.5,
timeout: 15
}
})
Using callbacks
Variable values are fetched asynchronously from the Leanplum servers. To use the latest values, get the Variable values through a callback. This also ensures proper handling during development and when you fetch variable values through forceContentUpdate
calls.
Leanplum.addVariablesChangedHandler(function() {
var variables = Leanplum.getVariables();
document.querySelector("#welcomeMessage").innerText = variables.welcomeMessage;
})
Updated about 5 years ago